How can I read CSV files interactively in Python?
Invoke the file dialog and select the CSV file as above.
Once you receive the CSV file, you can read it using the CSV module as usual.
import sc3
import datetime
import ctypes
import os
import csv
import win32gui, win32con
def get_open_file_name(title):
filter='csv\0*.csv\0'
customfilter='Other file types\0*.*\0'
fname, customfilter, flags=win32gui.GetOpenFileNameW(
InitialDir=project_file_path,
Flags=win32con.OFN_ALLOWMULTISELECT|win32con.OFN_EXPLORER,
File='', DefExt='csv',
Title=title,#
Filter=filter,
CustomFilter=customfilter,
FilterIndex=0)
return str(fname)
def get_shift_info():
filename=get_open_file_name("Open CSV File")
print(filename)
list=[]
with open(filename, encoding='utf8', newline='') as f:
csvreader = csv.reader(f)
for row in csvreader:
list.append(row)
print(row)
get_shift_info()#Your Operation
Load the Project File
File → Open Project File from GitHub
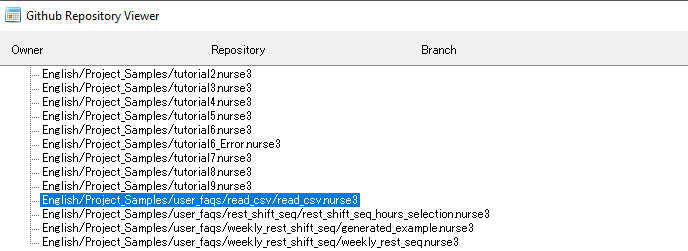